多态是指代码可以根据类型的具体实现采取不同行为的能力。如果一个类型实现了某个接口,所有使用这个接口的地方,都支持这种类型的值。
如果用户定义的类型实现了某个接口类型声明的一组方法,那么这个用户定义的类型的值就可以赋给这个接口类型的值。
go语言的接口采用鸭子类型的思想(如果走起路来像鸭子,那么它就是鸭子),实现时不需要显式的声明实现了什么接口。下面的Cat和Dog的实现是一个例子:
package main
import (
"fmt"
)
type Animal interface {
Eat()
Sleep()
}
type Cat struct {
name string
food string
}
func (c *Cat) Eat() {
fmt.Printf("%s eats %s.\n", c.name, c.food)
}
func (c *Cat) Sleep() {
fmt.Printf("%s sleep.\n", c.name)
}
type Dog struct {
name string
food string
}
func (d *Dog) Eat() {
fmt.Printf("%s eats %s.\n", d.name, d.food)
}
func (d *Dog) Sleep() {
fmt.Printf("%s sleep.\n", d.name)
}
func main() {
animal1 := &Cat{"cat", "fish"}
Eat(animal1)
Sleep(animal1)
animal2 := Dog{"dog", "pork"}
Eat(&animal2)
Sleep(&animal2)
}
cat eats fish.
cat sleep.
dog eats pork.
dog sleep.
main函数创建一个Animal类型的值, 并将animal1和animal2的地址传给了Eat和Sleep, 这会导致最终具体类型Cat和Dog的对应方法的执行, 因此这个Eat和Sleep函数可以同时执行Cat和Dog的行为
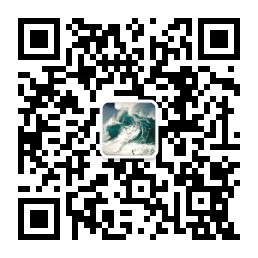
微信扫一扫,订阅我的博客动态^_^